Contents
- Introduction
- Using logger from shell
- Using logger from PHP
- Using logger from Python
- Sending realtime task progression
Introduction
All tasks can send output to the well known streams 'stdout' and 'stderr'. But sometimes, you want to send more informations for logging or debugging purpose. It is not desirable to send it to stdout as it overloads the output with debugging informations. Moreover, it will corrupt XML output if you are using it. Sending it to stderr could be a solution, but it might not be advisable either if it's not really some error output.
For these reasons, evQueue provides a special stream for logging informations. It is displayed in the 'log' tab of tasks details.
This stream is available on the descriptor 3 of any task. If the task is running over SSH, you must enable evQueue agent for this to work. To enable the agent, you must install it on the remote machines (see download page) and enable the agent in the task configuration.
Using logger from shell
If you are using shell scripts, using the logger is pretty straightforward. You just need to redirect your outputs to descriptor 3:
echo "This for evQueue logger" >&3
That's it! You should see this log in the dedicated tab of the task details.
Using logger from PHP
Using it from PHP is slightly more complicated: you have to use the special stream 'php://fd/3'. Open it and write with standard file access functions:
$logdesc = fopen('php://fd/3','w'); fwrite($logdesc, "This for evQueue logger\n");
You're good. As you can see this is not much more complicated.
Using logger from Python
For Python, you have to use the built-in posix module:
import posix; posix.write(3,"This for evQueue logger\n");
Sending realtime task progression
Another feature provided by evQueue logger is realtime task progression. The task can output its current completion status which will be displayed in realtime on the evQueue board.
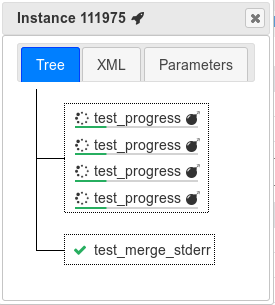
Of course, it's not always possible to compute this, but for tasks treating text input files (for example), this is pretty easy.
To send this information to evQueue, you have to use the logger stream and send progression (from 0 to 100) on one line precedeed by '%'. Here is a bash sample displaying progression over 10 seconds:
for((i=0;i<10;i++)) do echo "%$(($i*10))" >&3; sleep 1 done
Same output in PHP:
$logdesc = fopen('php://fd/3','w'); for($i=0;$i<10;$i++) { fwrite($logdesc,"%".($i*10)."\n"); sleep(1); }